Mastering APIs: A Simplified Guide for Product Managers (3/3)
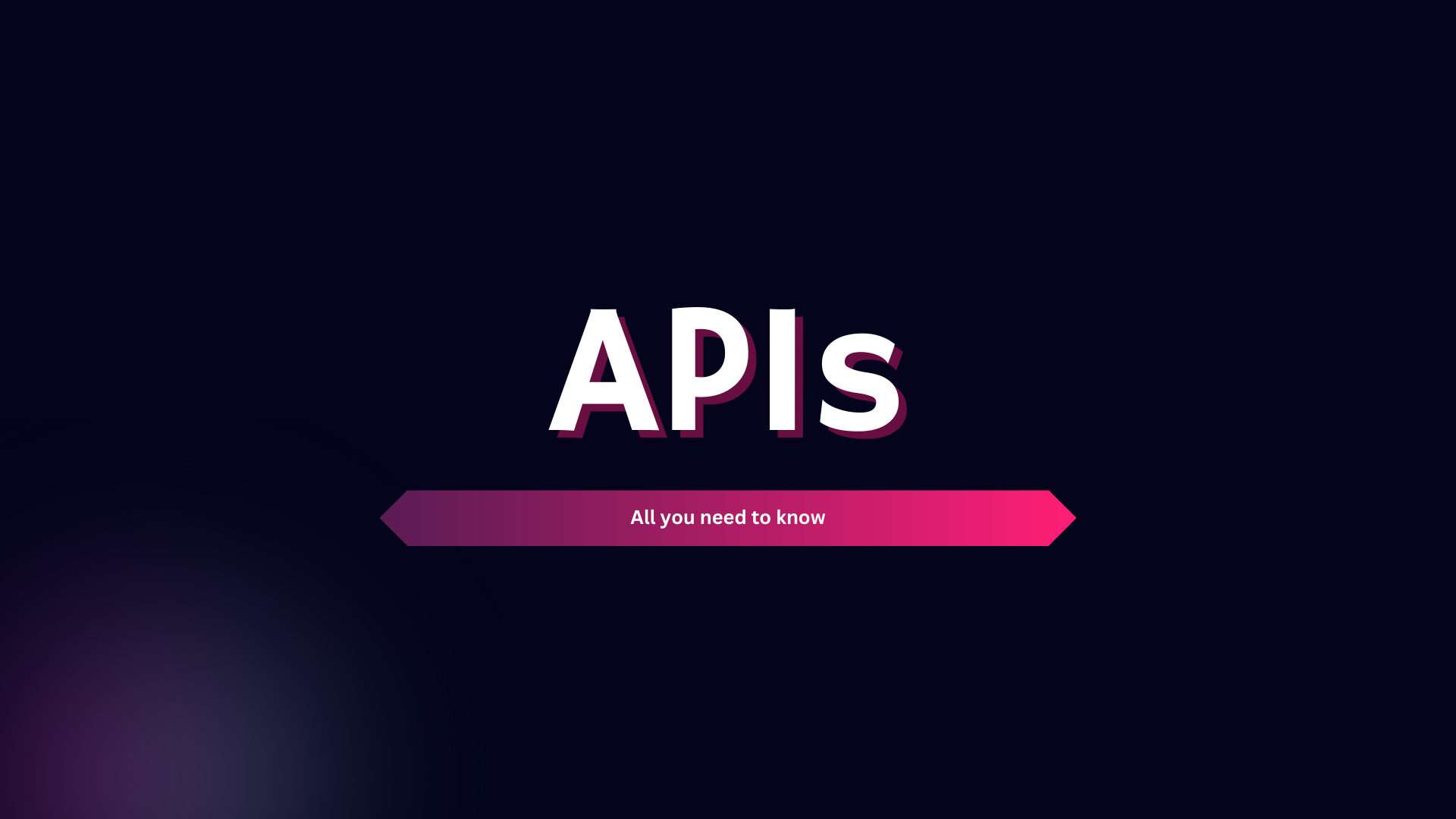
c. API server
Once the API client assembles the request, it sends it to the appropriate endpoint on the API server for processing. The API server is responsible for handling authentication, validating input data, retrieving or manipulating data from a database, and returning the appropriate response to the client.
- Role: The API server acts as an intermediary between clients (such as web or mobile applications) and the server-side resources or services.
- Function: It processes incoming requests, performs necessary operations (like data validation, business logic execution), and then returns the appropriate responses to the clients.
- Endpoints: It exposes endpoints (URLs) that clients can use to perform various operations like creating, reading, updating, or deleting data (CRUD operations).
- Security: Implements security features such as authentication (verifying user identity), authorization (ensuring users have permission to perform actions), and encryption (using HTTPS).
You must be wondering how Client, API Server, and database work together, right? Here we go:
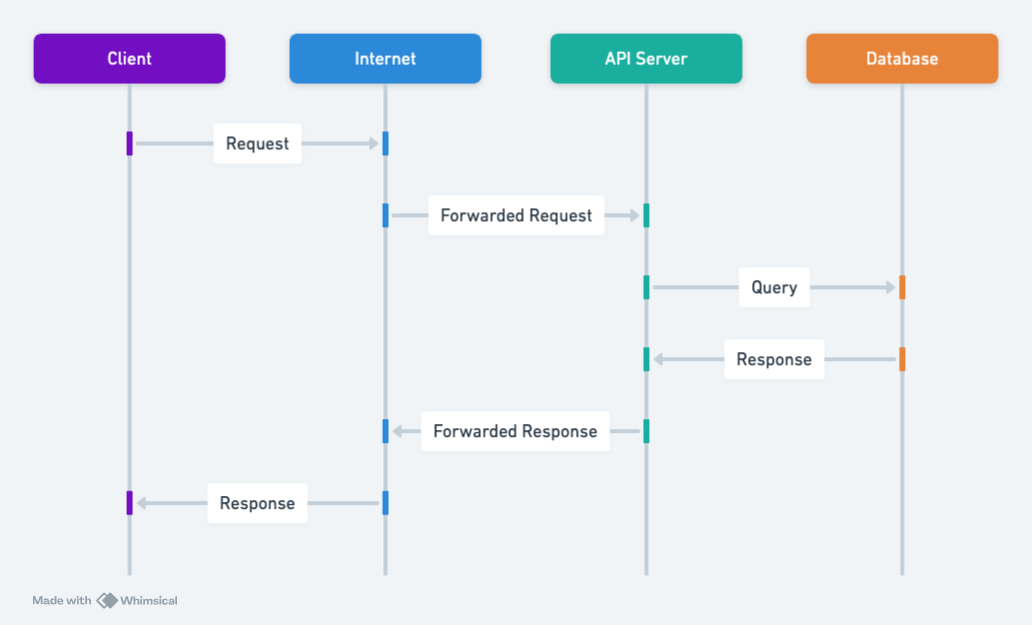
- Client Request: A client (e.g., a mobile app) sends an HTTP request to an API server to perform some operation, such as adding a new task to a to-do list.
- API Server Processing: The API server receives this request at a specific endpoint, processes it (validates the input, applies business logic), and determines what needs to be done.
- Database Interaction: If the operation involves data info, storage or retrieval, the API server will interact with the database. For example, to add a new task, the API server sends a query to the database to insert the new task data.
- Database Response: The database executes the query and returns the result to the API server, such as confirmation of the data being stored or the retrieved data requested by the client.
- Server Response: The API server then constructs a response based on the database's result and sends it back to the client.
Now you understand the relation between API Client, Server, and database?
Note: It’s important to note that while the database itself is not an API component, the API cannot function without it. Whereas the API server retrieves and manipulates application data based on a request, the database stores and organizes this data in a way that facilitates efficient retrieval and manipulation. The API server therefore acts as an intermediary between the API client and the database.
d. API response
An API response is simply the data sent back from an API server to the client after processing an API request. It conveys the outcome of the request, such as the success or failure of the requested operation and any relevant data or error messages. Here are the main components of an API response:
1. Status Line
The status line includes the HTTP version, a status code, and a reason phrase. It indicates the result of the request.
- HTTP Version: The version of the HTTP protocol (e.g., HTTP/1.1).
- Status Code: A three-digit code that indicates the result of the request.
- 2xx: Success (e.g., 200 OK, 201 Created)
- 4xx: Client errors (e.g., 400 Bad Request, 404 Not Found)
- 5xx: Server errors (e.g., 500 Internal Server Error)
- Reason Phrase: A brief description of the status code.
Example:
HTTP/1.1 200 OK
2. Headers
Headers are key-value pairs that provide additional information about the response. Common headers include:
- Content-Type: The media type of the response body (e.g.,
application/json
). - Content-Length: The size of the response body in bytes.
- Date: The date and time when the response was generated.
- Server: Information about the server handling the request.
Example:
Content-Type: application/json
Content-Length: 85
Date: Wed, 30 May 2024 12:00:00 GMT
Server: Apache/2.4.1 (Unix)
3. Response Body
The response body contains the actual data returned by the API. It is usually in JSON format, but it can also be in XML, HTML, or plain text. The body includes the requested data or error messages.
Example of a successful response body (JSON):
{
"id": 1,
"title": "Buy groceries",
"dueDate": "2024-05-28"
}
Example of an error response body (JSON):
{
"error": "Invalid input",
"message": "Title is required"
}
4. Common Components in a JSON Response Body
- Data: The main content or payload. It could be a single resource, a list of resources, or other relevant data.
- Metadata: Additional information about the response, such as pagination details.
- Errors: Information about any errors that occurred during processing.
Example of a Complete API Response
Imagine a client requests to retrieve a list of tasks from a to-do list API.
Request:
GET /api/tasks HTTP/1.1
Host: api.todoapp.com
Response:
HTTP/1.1 200 OK
Content-Type: application/json
Content-Length: 155
Date: Wed, 30 May 2024 12:00:00 GMT
Server: Apache/2.4.1 (Unix)
{
"data": [
{
"id": 1,
"title": "Buy groceries",
"dueDate": "2024-05-28"
},
{
"id": 2,
"title": "Call the bank",
"dueDate": "2024-05-29"
}
],
"metadata": {
"totalItems": 2
}
}
Few Miscellaneous concepts:
1- cURL:
cURL typically specifies the HTTP method and the endpoint (URL) along with any necessary headers, data, or options. Here's a basic example of how cURL can be used to make a GET request to an HTTP endpoint:
Here's an example of a POST request with cURL, including headers and data:
curl -X POST http://example.com/api -H "Content-Type: application/json" -d '{"key":"value"}'
In this example:
-X POST
specifies the HTTP method (POST).http://example.com/api
is the endpoint.-H "Content-Type: application/json"
sets the request header to indicate the data type being sent.-d '{"key":"value"}'
specifies the data to be sent in the request body.
cURL is a powerful tool for testing and interacting with web services, allowing you to customize your requests with various options and parameters.
2- URL, URI, URN
- URI: A general identifier for a resource (can be a URL or a URN). Example:
http://example.com/page
(URL) orurn:isbn:978-3-16-148410-0
(URN) - URL: A specific type of URI that locates a resource by its network address (https://www.example.com/index.html)
- URN: A specific type of URI that names a resource without indicating its location.
Ex-urn:oasis:names:specification:docbook:dtd:xml:4.1.2
3- API Key
- What it is: A unique identifier used to authenticate a client making a request to the API.
- Why it's important: It helps ensure that only authorized clients can access the API.
Example Usage:
GET /api/data HTTP/1.1
Host: api.example.com
Authorization: Bearer your-api-key-here
4- Rate Limiting
- What it is: A mechanism to control the number of requests a client can make to an API in a given period.
- Why it's important: It helps prevent abuse and ensures fair use of the API by all clients.
- Example:
- Limit: 100 requests per hour
5- OAuth
- What it is: An open standard for access delegation, commonly used for token-based authentication and authorization.
- Why it's important: It allows third-party applications to securely access server resources on behalf of a user.
- Example Usage:
- OAuth 2.0 tokens are used to access APIs without sharing user credentials.
6- Webhooks & Websockets
WebSockets
- What they are: WebSockets are a protocol for real-time, two-way communication between a client (e.g., a web browser) and a server.
- How they work: Once a WebSocket connection is established, the client and server can send messages to each other at any time without needing to reopen the connection.
- Use Cases: Ideal for applications that require constant, low-latency data exchange, such as live chat apps, online gaming, and real-time notifications.
- Example: A chat application where messages are sent and received instantly.
Note: This enables bi-directional communication, allowing both client and server to send messages independently and maintain a persistent connection, allowing continuous data exchange without reopening connections. This is best for real-time applications needing constant data flow, like chat apps or live updates.
Webhooks
- What they are: Webhooks are HTTP callbacks triggered by specific events in a system, sending real-time data to another system.
- How they work: When an event occurs, the source system sends an HTTP POST request to a predefined URL, notifying the receiving system of the event.
- Use Cases: Suitable for scenarios where one system needs to notify another about events, such as user registrations, payment processing, or content updates.
- Example: An e-commerce platform sending a webhook to a fulfillment service when a new order is placed.
Note: These are unidirectional, with the server pushing data to the client upon an event trigger and do not maintain a persistent connection; each event triggers a separate HTTP request. This is ideal for event-driven notifications where the receiving system needs to act on specific events, like automated emails or integrations between different services.
7- SDK (Software Development Kit)
- What it is: A collection of software tools and libraries designed to help developers create applications for specific platforms.
- Why it's important: SDKs simplify the integration of complex API functionality into applications.
- Example: Facebook provides an SDK for integrating its social login feature into apps.
8- Authentication vs. Authorization
- Authentication: The process of verifying the identity of a user or client.
- Example: Logging into an app using a username and password.
- Authorization: The process of determining what actions a user or client is allowed to perform.
- Example: Checking if a logged-in user has permission to access an admin panel.
9- Rate Limiting
- What it is: A technique to control the amount of incoming requests to an API.
- Why it's important: It prevents abuse, ensures fair usage, and protects the API from being overwhelmed.
- Example: An API might allow a maximum of 100 requests per minute per user.
10- Throttling
- What it is: Similar to rate limiting, it controls the rate of requests but typically applies more dynamically based on current load.
- Why it's important: It helps manage load and performance by slowing down clients when the system is under high demand.
- Example: Reducing the rate of API requests during peak times to prevent server overload.
11- CORS (Cross-Origin Resource Sharing)
- What it is: A security feature implemented by browsers to restrict how web pages can make requests to a different domain.
- Why it's important: It helps prevent security issues like cross-site scripting (XSS).
Example: An API server can use CORS headers to allow specific domains to access its resources:
Access-Control-Allow-Origin: https://example.com
These additional components and concepts are essential for understanding the full scope of API development, testing, and usage.
12- JSON (JavaScript Object Notation)
- What it is: JSON is a lightweight data-interchange format that is easy for humans to read and write, and easy for machines to parse and generate.
- Why it's important: It is widely used in APIs to format data due to its simplicity and compatibility with many programming languages.
Example: An API response in JSON format might look like:
{
"userId": 1,
"name": "John Doe",
"email": "john.doe@example.com"
}
13- XML (eXtensible Markup Language)
- What it is: XML is a markup language that defines a set of rules for encoding documents in a format that is both human-readable and machine-readable.
- Why it's important: XML is used in various APIs, especially older or enterprise-level services, for data interchange.
Example: An API response in XML format might look like:
<user>
<userId>1</userId>
<name>John Doe</name>
<email>john.doe@example.com</email>
</user>
14- Versioning
- What it is: Versioning is the practice of managing changes to an API by assigning version numbers to different iterations.
- Why it's important: It ensures backward compatibility and allows developers to introduce new features or changes without disrupting existing clients.
- Example: An API endpoint might include a version number in the URL, such as
https://api.example.com/v1/users
.
15- Load Balancer
- What it is: A device or software that distributes incoming network traffic across multiple servers.
- Why it's important: Ensures no single server becomes overwhelmed by distributing the workload evenly.
- Example: A website with high traffic might use a load balancer to distribute requests among multiple servers, improving performance and reliability.
16- API Sandbox
- What it is: A testing environment that simulates the behavior of a production API.
- Why it's important: It allows developers to test and experiment with API integrations without affecting live data or systems.
- Example: Many payment gateways provide sandbox environments where developers can simulate transactions and test their integrations.
17- API Documentation
- What it is: Comprehensive information about how to use an API, including endpoints, request and response formats, parameters, and authentication methods.
- Why it's important: Good documentation helps developers understand how to integrate with and use the API effectively.
- Example: Swagger and Postman provide tools for creating interactive API documentation.
That's it. You are a genius now! If you know this much about the APIs,
that's enough!
Now go to ChatGPT and ask
"Give me 30 MCQs about API. As a Product Manager I want to test my knowledge level".